Entries tagged "JavaScript"
Convert Plain Text to HTML List with Dreamweaver Object
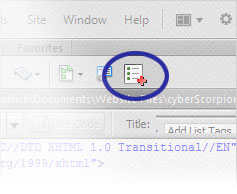
I commonly need to convert blocks of plain text into bulleted lists. The task involves highlighting each list item and enclosing it with <li> tags. The process doesn't take long to complete, but it could be streamlined. So I took the opportunity to create my first Dreamweaver Object. [Continue reading]
Check Array and Object Values in JavaScript with console.dir()
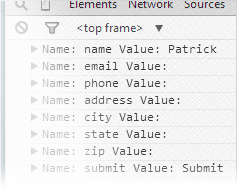
The alert() method in JavaScript is useful for quickly seeing what a variable contains as the program executes, but it has some limitations. It won't display the values stored in an array without creating a loop, for example. If you do create a loop, alert boxes can be aggravating since they each need to be confirmed separately. Luckily, there is another JavaScript method. [Continue reading]
Make Sure Things Are Working Correctly in JavaScript with the alert() Method
My usage of JavaScript can be a bit sporadic. There are times when I go months without writing a line of JavaScript code. So I find myself forgetting how to do certain things. It also doesn't help that most of my time is spent with PHP which adds to the confusion since the languages have different aspects to them. To help get my bearings, I've used JavaScript's alert() method to quickly see things like what value a variable contains. Let's look at a simplified example showing how I use alert(). [Continue reading]
Duplicate Log Entries Caused by the AddThis Widget
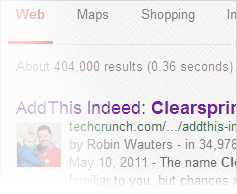
A search engine I develop was showing some odd behavior a few weeks ago. While making a few back-end enhancements, I noticed that many of the log entries were being duplicated. And they weren't the typical duplicates caused by a programming error. These entries were being entered after a 20 to 30 minute delay. Plus, the duplicates originated from a completely different IP address. Well, it turns out that the AddThis code I installed on the website was the culprit. [Continue reading]
Pre-Populate Forms that Are Hosted on Other Websites
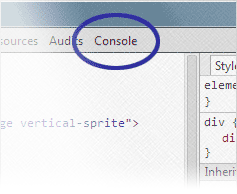
Lately, I've been more involved with submitting content to other organizations' websites. Getting indexed on those websites helps make the content more widely available. Since the information being submitted is already in a database or doesn't change from submission to submission, it would be nice if I didn't need to re-type or copy/paste everything. Luckily, there is a quicker way. [Continue reading]
Calculating the Distance between Zip Codes Using the Google Maps API – Part 2
Last week, we went over the basics for accessing the Google Maps API and calculating mileage between zip codes with Google's Distance Matrix service. The problem is that the service only lets us work with 25 origin and 25 destination points at a time. A custom solution is needed to go beyond that. [Continue reading]
Calculating the Distance between Zip Codes Using the Google Maps API – Part 1
A request came in recently to develop a feature for getting a list of organizations near a zip code provided by the user. They're looking for something very similar to a jobs website where you can narrow the search results to show only the ones within a 50 or 100 mile radius. The Google Maps API and Google's Distance Matrix service seem like great solutions for the problem. [Continue reading]
Why PHP_SELF Should Be Avoided When Creating Website Links
When looking for articles about PHP_SELF, it seems like most only refer to the dangers of using the variable with HTML forms. However, there are risks with using it in other parts of a website. For example, it may be tempting to use the variable within the href attribute for links. The problem is that those links become susceptible to Cross-Site Scripting (XSS). Let's take a closer look at the security vulnerability of PHP_SELF and a simple alternative to avoid the problem altogether. [Continue reading]
Generate Usernames with JavaScript: Working with Short Last Names
When generating usernames, one thing to consider is the length of the username. The code from last week's post may be problematic if you're looking for the username to be five characters or more and the user's last name is only two characters. After tacking on the first initial, you would only have three characters. So let's look at getting closer to the desired results. [Continue reading]
Using JavaScript to Dynamically Generate the Username within an HTML Form
Usernames are typically made up of some combination of the user's first and last name. If that's the case, the form used to create those usernames could be modified to take advantage of the data in the first and last name fields. Instead of making someone manually type the username, JavaScript could be employed to generate it automatically. [Continue reading]